python+flask 实现鲁迅名言查询系统
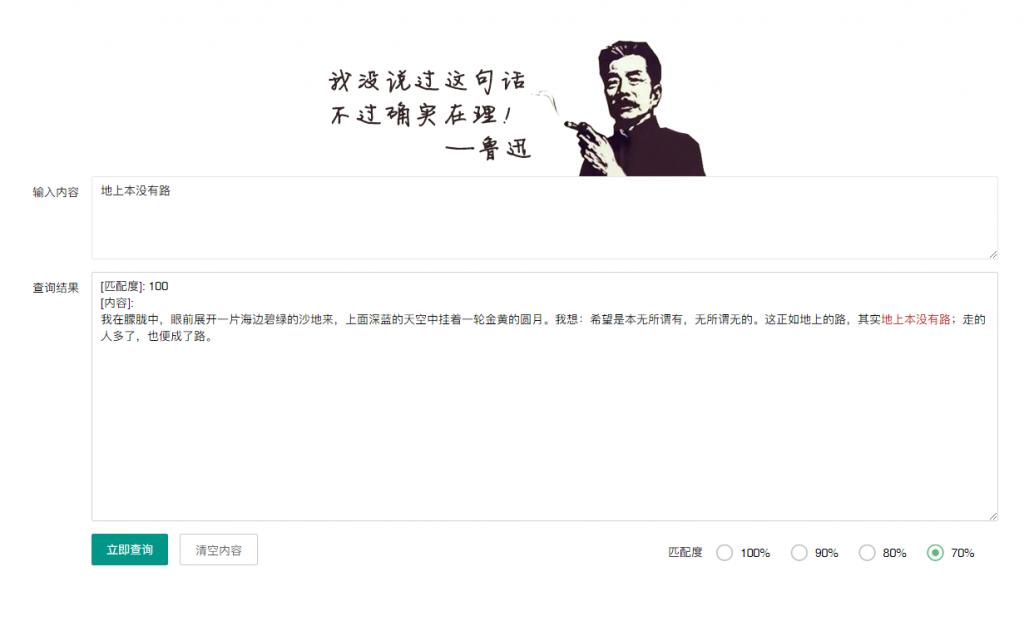
PY文件编写
from flask import Flask,render_template,request,jsonify
import requests,json,sys
from fuzzywuzzy import fuzz
from fuzzywuzzy import process
app = Flask(__name__)
#鲁迅名言查询页面路由配置
@app.route('/chaxun', methods=['GET'])
def chaxun():
return render_template('chaxun.html')
@app.route('/chaxun', methods=['POST'])
def inquiry():
paragraphs = []
with open('app/static/files/book.txt', 'r', encoding='utf-8') as f:
for line in f.readlines():
if line.strip():
paragraphs.append(line.strip('\n'))
data = json.loads(request.form.get('data'))
word = data['word']
pipei = data['pipei']
sentence = word
#匹配度
score_thresh = int(pipei)
matched = []
for p in paragraphs:
score = fuzz.partial_ratio(p, sentence)
if score >= score_thresh and len(sentence) <= len(p):
matched.append([score, p])
infos = []
for match in matched:
infos.append('<p>[匹配度]: %d<br/>[内容]:<br/> %s</p>' % (match[0], match[1]))
if not infos:
infos.append('未匹配到任何相似度大于百分之%d的句子.' % score_thresh)
return jsonify({"success": 200, "msg": "查询成功","res":infos})
if __name__ == '__main__':
# server = WSGIServer(('127.0.0.1',5000),app,handler_class=WebSocketHandler)
# server.serve_forever()
app.run(debug=True) #FLASK开启调试模式,使程序修改即时生效
HTML模板编写
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>鲁迅名言查询系统</title>
<link rel="stylesheet" href="../static/layui/css/layui.css">
<link rel="stylesheet" href="../static/style.css">
<script src="../static/jquery-3.4.1.min.js"></script>
<script src="../static/layui/layui.js"></script>
<style type="text/css">
.result p{ margin-bottom: 20px; }
</style>
</head>
<body>
<div style="width: 1200px; margin: 100px auto;">
<script>
layui.use('form', function() {
var form = layui.form;
form.render();
});
layui.use('layer', function(){
var layer = layui.layer;
});
</script>
<div style="text-align: center;"><img src="../static/images/lx.png"/></div>
<form class="layui-form">
<div class="layui-form-item layui-form-text">
<label class="layui-form-label">输入内容</label>
<div class="layui-input-block">
<textarea placeholder="请输入内容" class="layui-textarea txt" name="words"></textarea>
</div>
</div>
<div class="layui-form-item layui-form-text">
<label class="layui-form-label">查询结果</label>
<div class="layui-input-block">
<div class="layui-textarea result" style="height: 300px; width: 1090px; overflow-y:auto;">
</div>
</div>
</div>
<div class="layui-form-item" style="width: 40%; float: left;">
<div class="layui-input-block">
<div class="layui-btn fybtn" lay-submit lay-filter="*">立即查询</div>
<button type="reset" class="layui-btn layui-btn-primary clearbtn">清空内容</button>
</div>
</div>
<div class="layui-form-item" style="width: 40%; float: right; margin-top: -50px; margin-right: -30px;">
<label class="layui-form-label">匹配度</label>
<div class="layui-input-block">
<input type="radio" name="pipei" value="100" title="100%" lay-filter="test1">
<input type="radio" name="pipei" value="90" title="90%" checked lay-filter="test1">
<input type="radio" name="pipei" value="80" title="80%" lay-filter="test1">
<input type="radio" name="pipei" value="70" title="70%" lay-filter="test1">
</div>
</div>
</form>
</div>
<script type="text/javascript">
$(function(){
$('.fybtn').click(function(){
var word = $('.txt').val();
var pipei = $('input:radio:checked').val();
var data= {
data: JSON.stringify({
'word': word,
'pipei':pipei,
}),
}
var index = layer.load();
$.ajax({
type: 'POST',
url: '/chaxun',
data: data, // 这个data是要post的数据
success: function(data){ // 这个data是接收到的响应的实体
//g,表示全部选中。即之后再替换AllReplace这个值的时候会选中所有的'被替换内容'进行替换
//因为jquery的replace默认只替换第一个,所以先用正则表达式选出所有要替换的内容。
var AllReplace = new RegExp(word,"g");
//JSON.stringify 把JSON转成字符串
content=JSON.stringify(data['res']).replace(AllReplace,'<span style="color:#CC0000">'+ word +'</span>');
//去掉字符串里的 ","
var AllReplace2 = new RegExp('","',"g");
content2=content.replace(AllReplace2,'');
//去掉字符串里的 ["
content3=content2.replace('["','');
//去掉字符串里的 "]
var AllReplace4 = new RegExp('"]',"g");
content4=content3.replace(AllReplace4,'');
$('.result').html(content4);
layer.close(index);
}
});
});
});
//点击匹配
layui.use('form', function () {
var form = layui.form;
form.on('radio(test1)', function (data){
var word = $('.txt').val();
var pipei = $('input:radio:checked').val();
var data= {
data: JSON.stringify({
'word': word,
'pipei':pipei,
}),
}
var index = layer.load();
$.ajax({
type: 'POST',
url: '/chaxun',
data: data, // 这个data是要post的数据
success: function(data){ // 这个data是接收到的响应的实体
//g,表示全部选中。即之后再替换AllReplace这个值的时候会选中所有的'被替换内容'进行替换
//因为jquery的replace默认只替换第一个,所以先用正则表达式选出所有要替换的内容。
var AllReplace = new RegExp(word,"g");
//JSON.stringify 把JSON转成字符串
content=JSON.stringify(data['res']).replace(AllReplace,'<span style="color:#CC0000">'+ word +'</span>');
//去掉字符串里的 ","
var AllReplace2 = new RegExp('","',"g");
content2=content.replace(AllReplace2,'');
//去掉字符串里的 ["
content3=content2.replace('["','');
//去掉字符串里的 "]
var AllReplace4 = new RegExp('"]',"g");
content4=content3.replace(AllReplace4,'');
$('.result').html(content4);
layer.close(index);
}
});
});
});
//点击清空输入框
$('.clearbtn').on('click',function () {
$('.result').html('');
$('.txt').html('');
})
</script>
</body>
</html>
欢迎留下你的看法
共 0 条评论